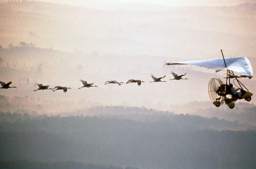
When writing data migrations, it can be useful to know the number of records that were updated / created. If you run your migration in a Rails console, ActiveRecord will print out the database response object like this for PostgreSQL:
2.1.5 :008 > DataMigrations::SomeMigration.call
=> #<PG::Result:0x0000000dc4edb8 @connection=#<PG::Connection:0x00000010e50348 @socket_io=nil, @notice_receiver=nil, @notice_processor=nil>>
You can get the number of rows affected by sending the #cmd_tuples
message to the result object like this:
query = <<-SQL
UPDATE some_table
SET some_field = TRUE
WHERE some_other_field > 1;
SQL
::ActiveRecord::Base.connection.execute(query).tap do |result|
puts "No. of records updated: #{result.cmd_tuples}"
end
Now you will get output that looks something like this:
2.1.5 :008 > DataMigrations::SomeMigration.call
No. of records updated: 3629
=> #<PG::Result:0x0000000dc4edb8 @connection=#<PG::Connection:0x00000010e50348 @socket_io=nil, @notice_receiver=nil, @notice_processor=nil>>
Also, I’ve found it helpful to run the migration in a staging environment using the Benchmark
module to get a feel for how long the migration will take in production like this:
Benchmark.realtime { DataMigrations::SomeMigration.call }