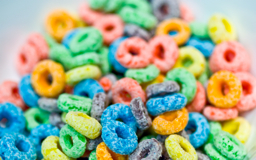
I prefer Ruby's Test::Unit
over RSpec, but one of the things I like about RSpec is the let
method. It allows you to create memoized helper methods in your tests. When I use Test::Unit, I usually mimic this behavior using a private helper method. Today I decided to see if I could write my own let
method that could be used with Test::Unit
.
Here's what I came up with:
class Test::Unit::TestCase
def self.let(name, &block)
ivar = "@#{name}"
self.class_eval do
define_method(name) do
if instance_variable_defined?(ivar)
instance_variable_get(ivar)
else
value = self.instance_eval(&block)
instance_variable_set(ivar, value)
end
end
end
end
end
Now instead of writing helper methods in your tests like this...
class EmployeeTest < Test::Unit::TestCase
def test_employee_has_an_email
assert_equal('jdoe@example.com', employee.email)
end
private
def employee
@employee ||= Employee.new('jdoe@example.com')
end
end
You can use "let" like this...
class EmployeeTest < Test::Unit::TestCase
let(:employee) { Employee.new('jdoe@example.com') }
def test_employee_has_an_email
assert_equal('jdoe@example.com', employee.email)
end
end