Hoboken
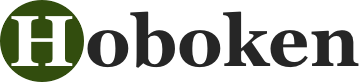
Basic Features
All projects generated with Hoboken have the following common features:
- sets up sinatra-asset-pipeline (non-API projects only)
- a
Rakefile
with tasks for running tests or specs - scripts for common tasks: installing dependencies, starting the development server, and starting a console (with racksh support)
- uses Puma as the default web server
- erubi templating; HTML escaping is on by default
- uses test-unit by default (RSpec can also be used, see the
--test-framework
option under the generate command) - Rack::Csrf is turned on by default
- environment variable support via the dotenv gem
- Procfile for foreman support; if foreman is not installed uses rackup instead
- code reloading via the rerun gem is enabled for the development environment
- pry-byebug for debugging
- custom Rack::Test helpers and assertions (or matchers for RSpec)
Project Structure
Projects are generated with the following structure:
Gemfile | Your app's dependencies |
---|---|
Procfile | Specifies the commands that are executed by the app on startup (foreman must be installed separately) |
README.md | A sample README |
Rakefile | Basic app tasks |
app.rb | The main application file |
config.ru | Rackup file |
/bin | Helper scripts for installing dependencies, starting the development server, etc. |
/config | Application configuration (Puma, etc.) |
/public | Static assets (i.e. css, js, images) |
/tasks | Various Rake task definitions |
/test or /spec | Basic unit test and test helper support |
/views | Your app's views (not present if --tiny option was used) |
The app.rb
file contains the main Sinatra application. If the --modular
option is used, the application will inherit from Sinatra::Base. Refer to the Sinatra documentation on classic vs. modular applications for more information.
General Command Reference
generate
Generate a new Sinatra app
$ hoboken generate [APP_NAME]
Options
[--ruby-version=RUBY_VERSION]
- Specify a Ruby version for the Gemfile. By default it will use the currently installed version.
[--tiny], [--no-tiny]
- Generate views inline; do not create /public folder.
[--type=TYPE]
- Architecture type (classic or modular). Default is classic.
[--git], [--no-git]
- Create a Git repository and make initial commit.
[--api-only], [--no-api-only]
- API only, no views, public folder, etc.
[--test-framework]
- Testing framework; can be either test-unit or rspec. Default is test-unit.
Rake Tasks
By default all newly generated projects will have the following Rake tasks available.
$ rake ci
- Runs tests (or specs) and any configured linting add-ons
$ rake test:all
- Run all tests (test-unit only)
$ rake test:integration
- Run tests for integration (test-unit only)
$ rake test:unit
- Run tests for unit (test-unit only)
$ rake spec
- Run all specs (RSpec only)
help
Describe available commands. If COMMAND is given, describe the specific COMMAND.
$ hoboken help [COMMAND]
version
Print version and quit.
$ hoboken version
Add On Command Reference
Airbrake
Support for Airbrake error reporting.
$ hoboken add:airbrake
Sets up Airbrake middleware.
GitHub Action
Github action that runs CI task
$ hoboken add:github_action
Adds a GitHub Action workflow that runs the ci
Rake task.
Heroku
Heroku deployment support.
$ hoboken add:heroku
Createa a .slugignore
file and configures $stdout
for Heroku logging. All Hoboken projects contain a Procfile
by default, which Heroku will use to start the application.
Internationalization
Internationalization support using sinatra-r18n.
$ hoboken add:i18n
Adds the sinatra-r18n gem to the project's dependencies and creates an i18n
folder with a sample translation file.
Metrics
Adds metrics utilities to project (flog, flay, simplecov).
$ hoboken add:metrics
Adds flog, flay, and simplecov to the project dependencies. Adds two rake tasks for running flog and flay. Modifies the test (or spec) rake tasks to run with simplecov. The coverage report is generated in the tmp/coverage
folder.
Omniauth
Authentication capability via the Omniauth gem.
$ hoboken add:omniauth
Running this command will prompt you to select an Omniauth provider and version. It will stub out 3 new handlers for Omniauth in app.rb
. In addition, it will create unit tests (or specs) for the stubbed out handlers.
Rubocop
Adds Rubocop support.
$ hoboken add:rubocop
ci
task is also modified to run Rubocop after tests/specs.
Sequel
Provides database support via the Sequel gem.
$ hoboken add:sequel
Adds the Sequel gem as a dependency. Also adds the sqlite3 gem for dev and test environments. You will need to specify your own database provider for production. Access to the database is handled via DATABASE_URL
which either needs to be exported as an environment variable or added to the project's .env
file. A config/db.rb
file is added to set up the database.
A db/migrate
folder is created for migrations. Two rake tasks for migrations are also created:
$ rake db:migrate
- Migrate the database to the latest version.
$ rake db:reset
- Perform migration reset (full erase and migration up).
The project's test_helper.rb
is adjusted to include a helper class for unit tests that touch the database. When a unit test class inherits from this, it will set up a test database and apply any migrations. Each test is run in the context of a transaction that will be rolled back after each test is run.
If RSpec is used, the project's spec_helper.rb
is adjusted instead. Any spec tagged with database: true
will be ran inside a database transaction that will be rolled back when the spec completes. Specs that use the rack: true
tag will also have this database rollback functionality.
Sidekiq
Adds Sidekiq support.
$ hoboken add:sidekiq
Sets up Sidekiq via a config/sidekiq.rb
file. Adjusts config.ru
to mount the Sidekiq UI. In production, the Sidekiq UI is protected via Basic Auth. Also adds a Sidekiq entry to the Procfile
.
Sinatra ActiveRecord
Provides database support via the Sinatra ActiveRecord gem.
$ hoboken add:active_record
Adds the sinatra-activerecord
gem as a dependency. Also adds the sqlite3 gem for dev and test environments. You will need to specify your own database provider for production. Access to the database is handled via DATABASE_URL
which either needs to be exported as an environment variable or added to the project's .env
file.
A db/migrate
folder is created for migrations. Rake tasks are also provided. Refer to the sinatra-activerecord
gem for more information.
The project's test_helper.rb
is adjusted to include a helper method for unit tests that touch the database. When a unit test class inherits from this, it will set up a test database and apply any migrations. Each test is run in the context of a transaction that will be rolled back after each test is run.
If RSpec is used, the project's spec_helper.rb
is adjusted instead. An around
block is added that handles the roll back for each spec example.
Travis CI
Basic Travis-CI YAML config.
$ hoboken add:travis
Sets up a minimal .travis.yml
file.
Turnip
Support for writing Turnip acceptance specs.
$ hoboken add:turnip
Sets up Turnip specs. Adds a Rake task to run them; also adds the task to the CI
task.
This add-on requires the use of RSpec.
Twitter Bootstrap
Twitter Bootstrap via the bootstrap gem.
$ hoboken add:twbs
Twitter Bootstrap support via the bootstrap
gem. Adjusts styles.scss
and app.js
to include bootstrap directives.
VCR
Support for the VCR gem.
$ hoboken add:vcr
Sets up VCR configured with webmock.